How to compile and run C and C++ code on Ubuntu desktop and server. If you have Ubuntu you already have g++ installed, which is C and C++ compiler. To check g++ version, simply launch your terminal and run the following command.
g++ --version
Install g++, C and C++ compiler on Ubuntu
In case it g++ is not installed on your system, run the following command to install g++
sudo apt update
sudo apt install g++
sudo apt install build-essential
g++ --version
Write your C hello world code, using your favorite text editor.
// file name: test.c
#include <stdio.h>
int main()
{
printf("Hello World\n");
return 0;
}
Compile C code with g++ with terminal
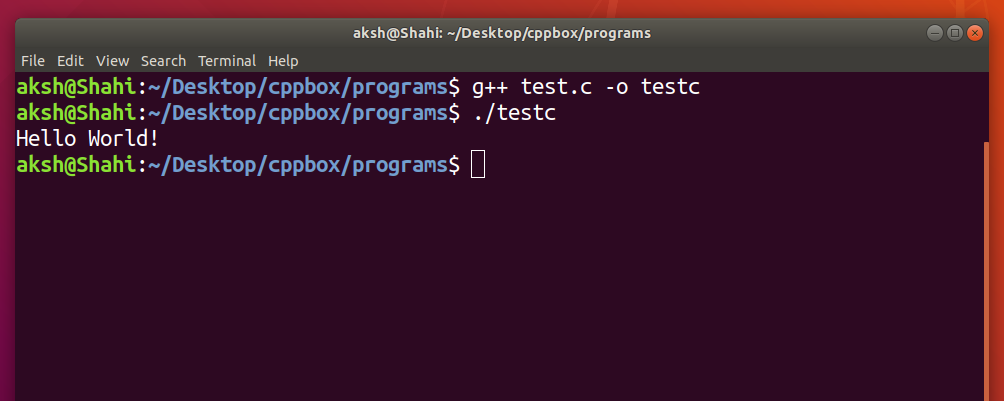
Navigate to test.c and test.cpp directory using your terminal and run the following commands.
g++ test.c -o testc
Here -o flag is given to output executable file name
Run / Execute C program with terminal
./testc
Write your C++ hello world code
// file name: test.cpp
#include <iostream>
using namespace std;
int main(){
cout << "Hello World\n";
return 0;
}
Compile C++ code with g++ in Terminal
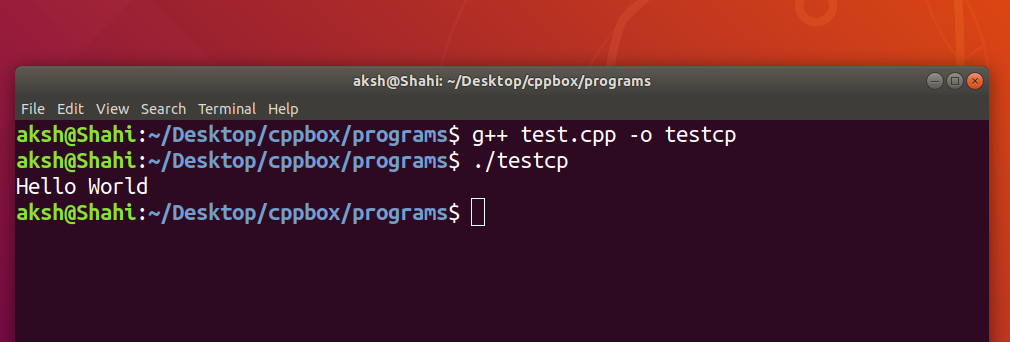
Navigate to test.c and test.cpp directory using your terminal and run the following commands.
g++ test.c -o testcp
Here -o flag is given to output executable file name
Run / Execute C++ program with terminal
./testcp
Install Make to compile C and C++ Faster
On Ubuntu Linux, you can install make tool to compile C and C++ code faster. Install make on Ubuntu with terminal.
sudo apt install make
Compile Code with Make
Compile C and C++ code with make, simply type make and file_name without extension like .c and .cpp
Run C and C++ Code with Terminal
./file_name