Hello there, this post will help you understand how some of WordPress image conversion plugins work behind the scenes. This is very simple straight forward process using php image manipulation library GD, which is a default library on almost every server with PHP.
GD can do a lot of things like image creation, editing, cropping, and etc. This post will only focus on converting png images to webp, which is a next generation of image for format as per Google page speed test tool. If you do not know webp image format increases the page load.
Let’s dive in right away, so here is the folder structure on my Windows 10 system, I am using xampp package for PHP & MySQL development environment setup.
This is my initial folder structure in htdocs folder
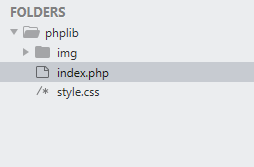
Here is the php code that converts png images into webp format in the same folder.
The first foreach loop uses glob() method to read each png image from the folder, imagecreatefrompng() method reads and verifies the png format and imagewebp() method converts png into webp format with 100% image quality.
The second foreach loop reads all the webp images and prints on the webpage.
<?php
// convert png to webp
foreach (glob('img/*.png') as $file) {
$im = imagecreatefrompng($file);
imagewebp($im, "$file".'.webp', 100);
}
// echo webp images on the webpage
foreach (glob('img/*.webp') as $webp) {
echo "<img src='".$webp."' />";
}
Folder structure after converting png images to webp format.
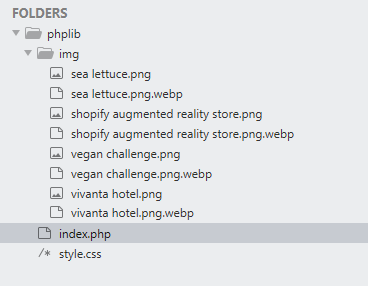
Suggestion: I should have removed png from from webp images but this is just experiment and I can do this in refactoring stage.
Convert PNG to JPG with PHP
To convert PNG images to JPG format with php, there are a few PHP inbuilt methods that we are going to use; the only requirement is that you need to have gd extension enabled on your server.
I have gd2 enabled in php-ini file
extension=gd2
Code to convert PNG to JPG format
<?php
// convert png to jpg
foreach (glob('img/*.png') as $file) {
$im = imagecreatefrompng($file);
imagejpeg($im, "$file".'.jpg', 80);
}
// echo jpg images on the webpage
foreach (glob('img/*.jpg') as $jpg) {
echo "<img src='".$jpg."' />";
}
Convert PNG images to JPEG in WordPress
There are many paid and free plugins out there that convert images from PNG format to JPEG or JPG. I am going to list a completely new plugin that works on both servers NGINX and Apache. Again your server must have gd2 enabled. In most cases it’s already enabled.

Convert PNG to WEBP in WordPress
You can convert PNG images into WEBP manually with PHP code above ; you just need to put the above png to webp example code in your theme functions file or you can create a WordPress plugin and installed it on all of WordPress instances and you are done.
Or you can use PNG to WEBP plugin from WordPress repository; some are free and some are paid. I have one free plugin that converts PNG to webp format but does not serve webp images onto the web browser.
To convert PNG images to WEBP format, I used the following WordPress plugin.
This plugin is completely free and there is no limit yet. Remember there is no undo button. Once it converts to webp format it’ll there on your server and new uploads will also be converted, if you don’t deactivate it.
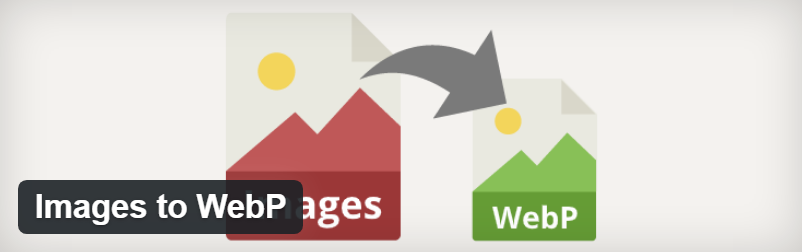
Serve WEBP images in WordPress
To server webp image format in WordPress there are a few paid and free plugins that you can use.
I have used EWWW image optimizer plugin that pulls webp images from the server and serves to the web browser client.
