Here is a quick approach to print pyramid patterns with JavaScript. It’s a simple and easy approach that anyone can easily understand and try on their own. I am taking rows value in every for loop in all the function code blocks.
Increasing Pyramid
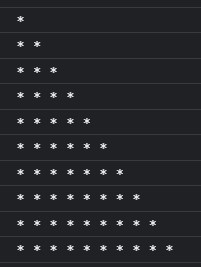
// increasing pyramid
function incPyra(rows){
for(let i = 0; i <= rows; ++i){
let pyramid = "* ".repeat(i)
console.log(pyramid)
}
}
incPyra(10)
Decreasing Pyramid
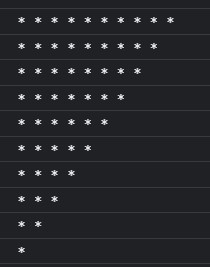
// Decreasing pyramid
function decPyra(rows){
for(let i = rows; i >= 1; --i){
let pyramid = "* ".repeat(i)
console.log(pyramid)
}
}
decPyra(10)
Opposite Side Increasing Pyramid
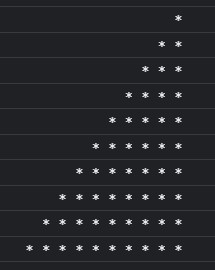
// opposite side increasing pyramid pattern with JavaScript
function rpyra(rows){
for(let i=0; i<=rows; i++){
let spaces = " ".repeat(rows-i)
let stars = "* ".repeat(i)
console.log(spaces + stars)
}
}
rpyra(10)
Opposite Side Decreasing Pyramid
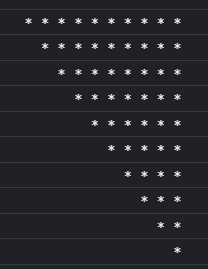
// opposite side decreasing pyramid pattern with JavaScript
function lpyra(rows){
for(let i=0; i<=rows; i++){
let star = '* '.repeat(rows-i)
let space = " ".repeat(i)
console.log(space+star)
}
}
lpyra(10)
Upside Down Pyramid
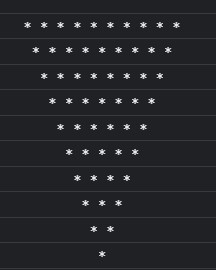
// upsite down pyramid
function usdPyramid(rows){
for(let i =0; i<=rows; ++i){
let stars1 = "* ".repeat(rows-i)
let spaces1 = " ".repeat(i)
console.log(spaces1 + stars1)
}
}
usdPyramid(10)
Pyramid Pattern
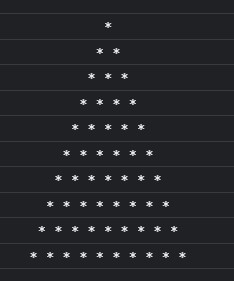
// pyramid
function pyramid1(rows){
for(let i = 0; i<=rows; i++){
let spaces = " ".repeat(rows-i)
let stars = "* ".repeat(i)
console.log(spaces+stars)
}
}
pyramid1(10)
Contents
show