This post will help you understand how to use PHP while loop to traverse and display MySQL table data in HTML. I have two tables in my MySQL database, named categories and brands that I want to view on html/php pages using PHP while loop.
I assure that you have established your MySQL database connection in your PHP project and now you want to traverse the table rows and columns in your HTML/PHP file.
I am doing an eCommerce project with PHP and MySQL using mysqli to understand the process.
My PHP work environment is Windows 10 Pro and I am using XAMPP for simplicity.
I am using phpmyadmin to manage my MySQL database. Here are my tables in my MySQL database.
Database Tables
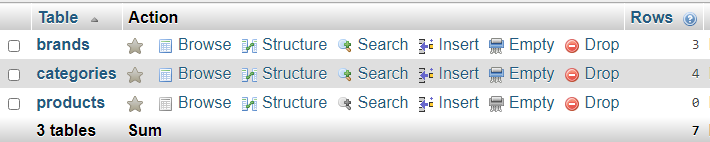
brands table structure

categories table structure

products table structure

Before you start writing PHP while loop to retrieve data from your MySQL server, make sure you have established database connection.
I have already established database connection on the top of index.php file.
PHP while loop for brands
Let me walk you through the code below. $brandsql variable holds all the brands table row from the database, which we are going to use to perform mysqli_query in the next line with $con variable which holds all the database connection credentials. You can put your mysql commands directly in the mysqli_query method but this is how people prefer doing it.
mysqli_query() method requires two parameters database connection and mysql command, which we have already given in form of php variables.
mysqli_query() method returns table row in form os both associative array and numeric array.
We are going to use PHP while loop to retrieve all the rows from the database table in a local variable called $barnd, I am using mysqli_fetch_assoc() method which takes an argument, which is result of mysqli_query() method. Also, mysqli_fetch_assoc() returns an associative array of values from each table row.
Now to access the database table values, we just need to type the table column name in square brackets of mysqli_fetch_assoc() return variable, which is $brand in our case.
One thing to remember that we cannot perform brand[“brand_name”]; directly in echo string or HTML, we need to first assign table row values to local variables and then use them in PHP echo method or HTML.
<h2>Brands</h2>
<nav class="secondary-menu">
<ul>
<?php
$brandsql = "SELECT * FROM brands";
$brand_row = mysqli_query($con, $brandsql );
while ($brand = mysqli_fetch_assoc($brand_row)) {
$bname = $brand["brand_name"];
echo "<li> <a href='$bname'>$bname</a></li>";
}
?>
</ul>
</nav>
Download PHP 7 eBook For Free
This is how it is showing in the HTML view.
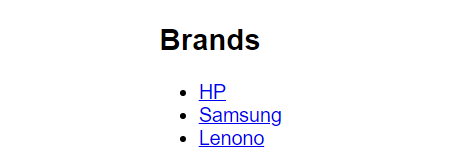
PHP while loop for categories
Here is another example of PHP while loop to retrieve table data from MySQL database.
<h2>Categories</h2>
<nav class="secondary-menu">
<ul>
<?php
$catsql = "SELECT * FROM categories";
$cat_row = mysqli_query($con, $catsql );
while ($cat = mysqli_fetch_assoc($cat_row))
{
$cname = $cat["cat_name"];
echo "<li> <a href='$cname'>$cname</a></li>";
}
?>
</ul>
</nav>
Here is how it shows in HTML view.
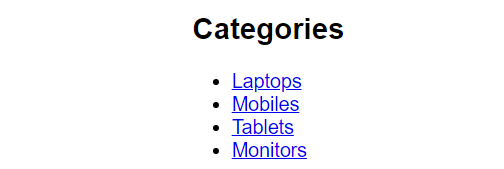