Create the Node using C++ class
It is a c++ singly linked list program using class and methods. This tutorial covers Node creation, Node insertion, and traversing the Node values.
#include <iostream>
using namespace std;
class Node {
public:
int data;
Node* next;
};
Declare the Link Class
class Link
{
public:
Node* head;
Link();
~Link();
void insertNode();
void displayNode();
};
class constructor and destructor in c++
Link::Link()
{
head = NULL;
}
Link::~Link()
{
}
class Link methods implementations
Insert New Node in Singly Linked List
void Link::insertNode() {
int length;
cout << "Enter Length: ";
cin >> length;
int value;
for (int i = 0; i < length; i++)
{
cout << "Enter Value # " << i + 1<<": ";
cin >> value;
Node* temp;
temp = new Node;
temp->data = value;
temp->next = head;
head = temp;
}
};
Display Node Values in Singly Linked List
void Link::displayNode() {
Node* temp = head;
cout << "Your List is\n";
while (temp != NULL)
{
cout << temp->data << " ";
temp = temp->next;
}
cout << endl;
};
Calling Link class methods in main function
int main() {
Link l;
l.insertNode();
l.displayNode();
return 0;
}
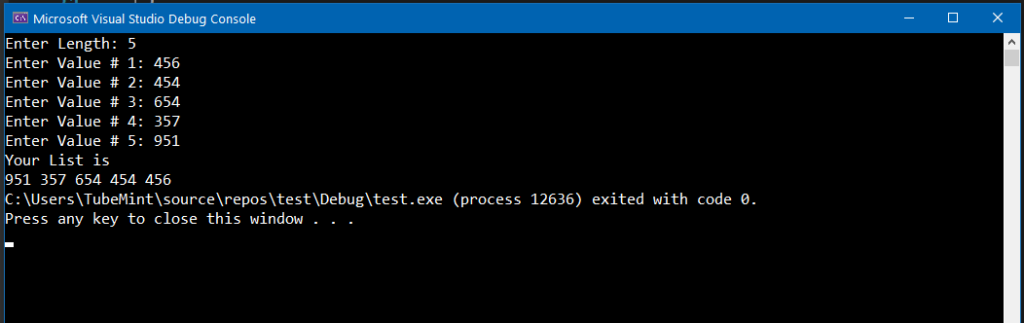
Here is the Singly Linked List C++ code sample
#include <iostream>
using namespace std;
class Node {
public:
int data;
Node* next;
};
class Link
{
public:
Node* head;
Link();
~Link();
void insertNode();
void displayNode();
};
Link::Link()
{
head = NULL;
}
Link::~Link()
{
}
void Link::insertNode() {
int length;
cout << "Enter Length: ";
cin >> length;
int value;
for (int i = 0; i < length; i++)
{
cout << "Enter Value # " << i + 1<<": ";
cin >> value;
Node* temp;
temp = new Node;
temp->data = value;
temp->next = head;
head = temp;
}
};
void Link::displayNode() {
Node* temp = head;
cout << "Your List is\n";
while (temp != NULL)
{
cout << temp->data << " ";
temp = temp->next;
}
cout << endl;
};
int main() {
Link l;
l.insertNode();
l.displayNode();
return 0;
}
Contents
show
Yo, thank you man it was very easy to understand. Much love.